class: center, middle, inverse, title-slide # Python基础 ## 🌱 ### 吴燕丰 ### 江西财大,金融学院 ### 2020/09/17 --- ### 标准数据类型 .pull-left[ 不可变数据(immutable): - Number(数字) - String(字符串) - Tuple(元组) ] -- .pull-right[ 可变数据(mutable): - List(列表) - Dictionary(字典) - Set(集合) ] --- ### Number(数字) ```python a, b, c, d = 20, 5.5, True, 4+3j print(type(a), type(b), type(c), type(d)) ``` ``` ## <class 'int'> <class 'float'> <class 'bool'> <class 'complex'> ``` .pull-left[ ```python 5 + 5 ``` ``` ## 10 ``` ```python 4.3 - 2 ``` ``` ## 2.3 ``` ```python 3 * 7 ``` ``` ## 21 ``` ```python 2 / 4 ``` ``` ## 0.5 ``` ] .pull-right[ ```python 2 // 4 ``` ``` ## 0 ``` ```python 17 % 3 ``` ``` ## 2 ``` ```python 2 ** 5 ``` ``` ## 32 ``` ] --- ### String(字符串) ```python str = 'JXUFE' print(str); print(str[0:-1]) ``` ``` ## JXUFE ## JXUF ``` ```python print(str[0]); print(str[2:]) ``` ``` ## J ## UFE ``` ```python print(str * 2); print(str + ' School of Finance') ``` ``` ## JXUFEJXUFE ## JXUFE School of Finance ``` --- ### Tuple(元组) ```python tuple = (1, 'two', '三') print(tuple); print(tuple[0]) ``` ``` ## (1, 'two', '三') ## 1 ``` ```python print(tuple[:1]); print(tuple[1:]) ``` ``` ## (1,) ## ('two', '三') ``` ```python print(tuple * 2); print(tuple + tuple) ``` ``` ## (1, 'two', '三', 1, 'two', '三') ## (1, 'two', '三', 1, 'two', '三') ``` -- <hr> <span style="color: yellow; background-color: gray;"> 修改元组元素的操作是非法的 </span> ```python tuple = (1, 'two', '三') tuple[0] = 100 ``` --- ### list(列表) 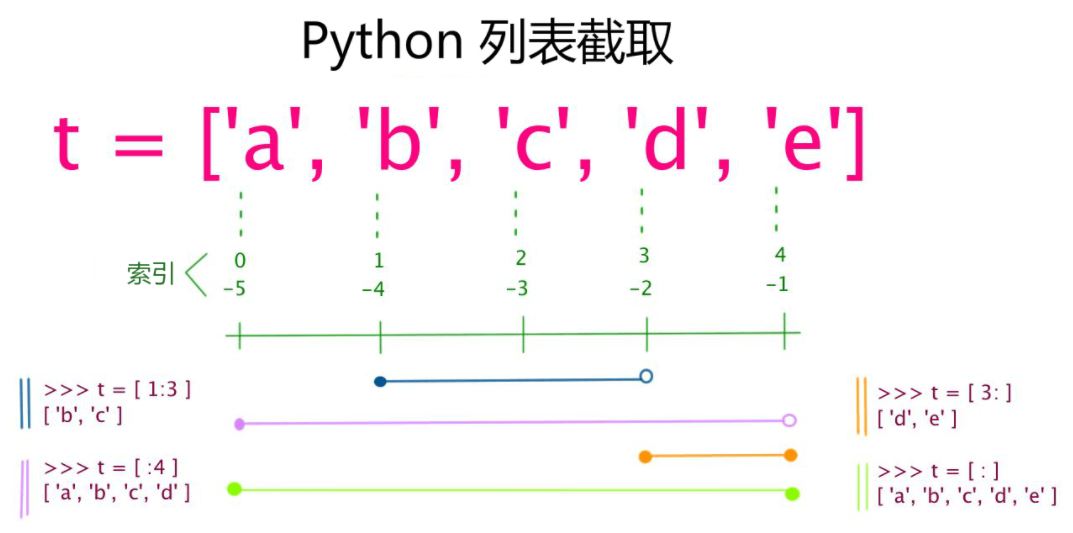 **左闭右开**:[n, m) .footnote[ 图片来源:https://www.runoob.com/python3/python3-data-type.html ] --- ### Dictionary(字典) ```python dict = {} dict['姓'] = '吴' dict['名'] = '燕丰' d2 = {'author': 'Yanfeng Wu', 'site': 'www.upjian.com'} print('姓: ' + dict['姓'] +';名: '+ dict['名']) ``` ``` ## 姓: 吴;名: 燕丰 ``` ```python print(d2) ``` ``` ## {'author': 'Yanfeng Wu', 'site': 'www.upjian.com'} ``` ```python print(d2.keys()) ``` ``` ## dict_keys(['author', 'site']) ``` ```python print(d2.values()) ``` ``` ## dict_values(['Yanfeng Wu', 'www.upjian.com']) ``` --- ### Set(集合) ```python A = set('abcd'); B = {'c', 'd', 'e', 'f'} print(A); print(B) ``` ``` ## {'a', 'b', 'c', 'd'} ## {'f', 'e', 'c', 'd'} ``` ```python print(A - B) ``` ``` ## {'a', 'b'} ``` ```python print(A & B) ``` ``` ## {'c', 'd'} ``` ```python print(A ^ B) ``` ``` ## {'e', 'a', 'b', 'f'} ``` --- ### 字符串的内建函数(built-in)函数 .pull-left[ - capitalize() - center(width, fillchar) - count(str, beg=0, end=len(string)) - isdigit(); isalpha() - join(seq) - replace(old, new [, max]) - split(str="", num=string.count(str)) - splitlines([keepends]) - zfill (width) - isdecimal() ] .pull-right[ - find(str, beg=0, end=len(string)); rfind(str, beg=0,end=len(string)) - ljust(width[, fillchar]); rjust(width,[, fillchar]) - lstrip(); rstrip(); strip([chars]) - endswith(suffix, beg=0, end=len(string));<br> startswith(substr, beg=0,end=len(string)) - maketrans() - translate(table, deletechars="") ] -- ```python 'JXUFE'.center(40, '♠') ``` ``` ## '♠♠♠♠♠♠♠♠♠♠♠♠♠♠♠♠♠JXUFE♠♠♠♠♠♠♠♠♠♠♠♠♠♠♠♠♠♠' ``` ```python '♣'.join(['J','X','U','F','E']) ``` ``` ## 'J♣X♣U♣F♣E' ``` --- ### 作业一 **最后一个单词的长度**: 请写代码计算出 [顺丰控股2019年年度报告(英文版).PDF](https://www.sf-express.com/cn/sc/download/20200701-IR-EN-2019-2.PDF) 第12页文本中最后一个单词的长度。 [作业一素材.txt](作业一素材.txt) 注意:不考虑页脚内容(顺丰控股股份有限公司 S.F. HOLDING CO., LTD.) --- ### 作业一 附加题 **最后一个句子的长度**(<span style="color:blue;">附加题,选做</span>): 请写代码计算出 [顺丰控股2019年年度报告.PDF](https://www.sf-express.com/cn/sc/download/20200324-IR-RPT-1-2019.PDF) 第13页文本中最后一个句子的长度。 [作业一附加选做题素材.txt](作业一附加选做题素材.txt) 注意:不考虑页脚内容(顺丰控股股份有限公司 S.F. HOLDING CO., LTD.) -- ### 使用open()函数 **提示:**可使用如下代码将文本读入内存: ```python # 须先将素材文件打开并保存至代码文件夹 f = open('作业一附加选做题素材.txt', 'w', encoding='utf-8') txt = f.read() f.close() ``` .footnote[ 如果运行报错,见 [解决方案](../../application/current_working_dir_explained/current_working_dir_explained.html#2)。 ] --- ### Just Code It .pull-left[ 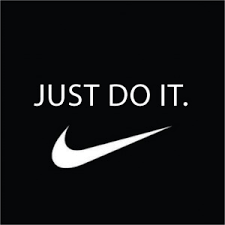 ] .pull-right[ 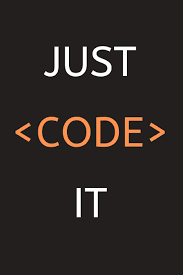 ]